Ultrawide monitors have been hyped since they came out, yet only a small fraction of people have actually experienced them. In this article I try to express my initial impressions with you.
The omnirole nature of C# and why it’s a top language
2/6/2024, 7:45:36 AM | by Tigrish | 2523 views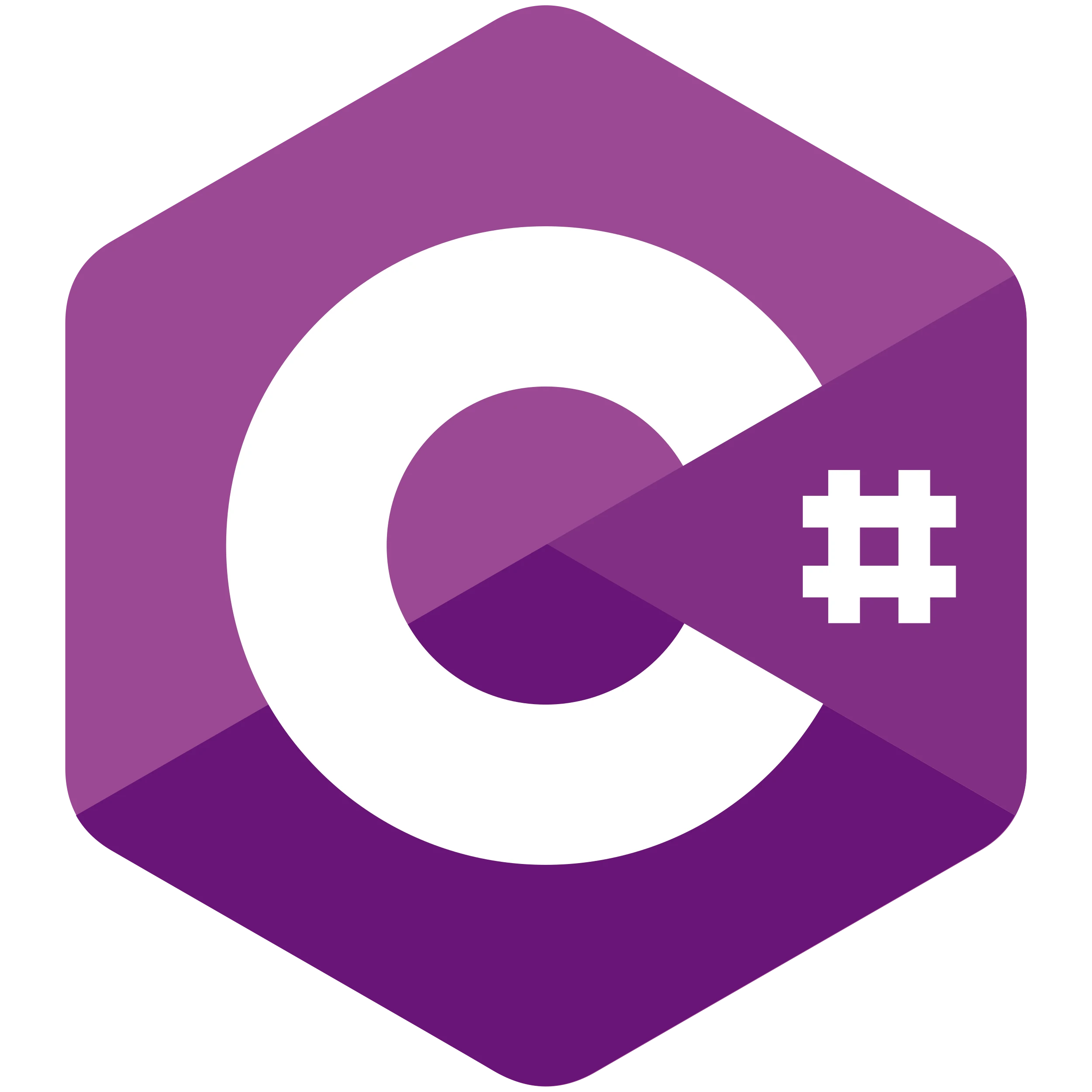
C# has enabled multitudes of performant and reliable applications to run for decades. A mature ecosystem, a huge class library and fun to write syntax make it a solid contender for the top league.
The basics
C# is a cross platform language that compiles to bytecode which runs on the .NET runtime, it is primarily object oriented but allows for a few functional/procedural elements that help speed up and simplify your code and architecture. It enables quick development through its C-like syntax while being high-level, fully type safe at both runtime and compile time. There are a ton of built-in libraries that cover most of the primary use cases and it has a rich ecosystem.
You can build many types of apps with it including web, desktop, mobile, games and embedded. It runs on Windows, Linux and MacOS, with some unofficial ports that handle the embedded aspect of it.
Whilst completely garbage collected, it maintains high performance and includes the tools you need to easily prevent unnecessary allocations if your app requires extracting the maximum performance.
The .NET runtime that C# runs on also supports other languages such as F# and Visual Basic, but more on those in another article.
My experience with it
I’ve worked almost exclusively with C# for everything but for making web frontends. It is super versatile and provides support with their ORM called Entity Framework (article coming soon) for most SQL databases such as PostgreSQL, MySQL, MSSQL and Oracle.
Everything is easy, from creating a project using a template to adding a library (called a nuget package), linking other projects and writing the code. I won’t dive into the specifics of the syntax in this article as there are many examples on the internet but rather a high-level overview of this ecosystem.
The syntax is super simple but provides many quality of life features such as data records, switch expressions, nullability checks and expressions, async, LINQ, easy dependency management and a huge class library included that covers most of what you need, just to name a few.
The power of async
Multithreading is always complex and resource intensive at scale. Asyncronous code allows you to create tasks that will run in the background. These tasks do not actually spawn a thread but are picked up by a scheduler that will orchestrate them based on available worker threads. When using the await
keyword inside an async
task, the scheduler may delegate IO work to the operating system or other relevant hardware, freeing up the worker thread for another task to take over while the first is awaiting its data.
Asynchronous programming is very powerful and most of C# first and third party libraries expose an async API. It's very easy to get into and provides noticeable performance uplifts, especially at scale.
The runtime
C# is a managed language, meaning that you won’t require interacting with the system or low-level code 99% of the time. It comes in two variants, .NET runtime managed and AOT (Ahead Of Time) compilation.
Runtime managed code
This is the classic and preferred way to compile and run your applications, it compiles your C# code into bytecode called MSIL and asks the runtime to interpret it can do a final runtime compilation using JIT before executing the logic. JIT allows for platform and context specific optimizations done on the host machine allowing for better performance in some cases. The first call to a method will usually take longer to execute, but all subsequent calls will run very fast.
A prime example of runtime optimization is query expressions using LINQ (Language integrated query) inside of Entity Framework, these expressions will get further optimized and result in optimal pre-generated SQL to speed up your queries. A whole lot more happens under the hood which I won’t talk about in this article.
The downside of runtime managed code is that you need an instance of .NET installed or packed in the executable which increases the bundle size quite a bit, but can be reduced using trimming in most cases. An app that includes the runtime can range from 20 MB to 60 MB depending on trimming options, excluding user code.
Ahead Of Time compilation
This is a newer approach which takes C# code and compiles it directly to machine native code, such as x86_64 Windows or aarch64 Linux. This method usually provides faster application startup time and improves performance in a lot of cases. Sadly, AOT foregoes any runtime optimizations and it can be hard to use certain libraries due to the excessive use of code trimming. A runtime is not needed to run this program and the final executables will be much smaller in size.
Making web applications
ASP.NET is the framework Microsoft provides for .NET, it includes around a dozen of sub-frameworks that achieve specific tasks. My personal favorites are Web API and Blazor. Below are some explanations of the major sub-frameworks:
Web API
A super versatile HTTP API system that uses the controller pattern, it allows for well organized code, dependency injection, session scopes and extensible by middleware. There is another variant called Minimal API which forgoes the controller aspect and lets you code endpoints procedurally, it is also AOT friendly. Performance is top tier and can be made even better using the async pattern.
It can interact with any client-side application that uses HTTP, such as browser SPAs and native applications. ASP.NET Web API is my go-to framework for making a backend.
MVC
MVC has fallen a bit out of fashion in the last few years due to the rise of SPA frameworks like React, Vue.js, Angular, etc… It provides full server-side rendering with all the benefits of Web API and has one of the best HTML templating engines ever made. Client-side reactivity needs to be made using Javascript, but you can easily use Typescript as well using pre-compilers.
Although it’s old, it is still a very performant system and a lot of jobs require it due to legacy applications. It is useful to at least know the basics if you get into C#
Blazor
Many people strongly dislike Javascript and maintaining an app that has a backend in C# and a frontend in JS/TS can increase the workload quite a bit. Hence, we got ASP.NET Blazor, a reactive UI templating engine that runs on C# and allows to write frontends almost exclusively using C#.
There are 2 variants of Blazor, hybrid and WASM.
Hybrid is a server side rendered application that is SEO friendly and loads fast while enabling interactivity, and downloads the full client-side app in the background. During the first load the code will run server-side using websockets and upon future sessions will run client-side using WASM.
The WASM variant is a client-side only app that downloads compiled C# code into the browser and runs it locally. The initial bundle size can be large in comparison to JS frameworks, but it performs quite well and is a definite recommendation if your web app does not require SEO optimization. I wrote an article about Blazor WASM here
Both variants provide a very good developer experience and function very well. They also both support Javascript interop to access certain APIs if need be.
Others
You are not limited to what is listed above, as you can use gRPC, SignalR/Websockets or even raw sockets. C# is a language that has the ability to adapt to almost any situation and probably has a well maintained library for what you need.
Native desktop and mobile
.NET provides a few first and third party frameworks for making UI applications that run natively on the host machine. Some use native controls and others use custom drawing libraries, here are a few of them:
AvaloniaUI
A personal favorite, it's an open source cross platform UI framework that works on MacOS, Linux, Windows that recently started supporting Android and iOS. It uses SkiaSharp to draw the UI elements and tries to maintain some level of compatibility with WPF for easy porting. Fully open source and free, very easy to write and supports the MVVM pattern by using ReactiveUI or MVVM Toolkit. It primarily uses XAML markup for defining controls. You can also port it to WebAssembly and run it from a browser, it is expected to have tvOS support in the future.
MAUI
Multiplatform App UI is made by Microsoft themselves. Based on the now deprecated Xamarin, it promises to be the next big UI framework that everybody should use to make cross platform apps, however, it doesn't support linux (yet). Microsoft seems invested in expanding on this project but it has been riddled by a lot of bugs as it is newer (made in 2022). However, the MAUI team, although small, has been very hard at work this past year and fixed a ton of bugs and it is progressing at a steady pace. I always recommend checking it out but be very mindful of using it in production before appropriate testing as it is still immature. It too uses XAML as the primary control definition .
WPF
A legacy but feature complete UI framework made by Microsoft in 2006. While it works well, it runs only on Windows and requires third party control libraries to look good and also uses XAML. If you have the option, use AvaloniaUI or MAUI.
Uno Platform
Uno is a third party framework like Avalonia which supports Windows, MacOS, Linux, Android, iOS and WebAssembly. I haven't tried it but it is quite promising based on received feedback.
WinForms
Winforms is not recommended for any new project, it is an outdated framework that is less scalable than WPF or Avalonia. Since it uses a drag & drop designer it may seem attractive to new C# coders, and it will run fine at first, but anyone with solid experience will tell you to stay away from it. It has however gotten a few updates with the .NET Core version that still make it viable in a serious environment.
Embedded applications
It is possible to create embedded app on low-resource systems using .NET NanoFramework. It works primarily on RISC-V ESP32 boards. Last I checked it supported neither generics nor async.
Game development
C# is widely used by game engines, it tends to lag behind C++ in performance when not properly optimized but most indie games will never be slowed down by it. As long as you are mindful of allocations and avoid unnecessary calls performance will likely not be impacted. Below are a few engines that allow the use of C# for game logic:
- Unity 3D
- Flax Engine
- Unigine
- Godot
- Monogame
- FNA (previously XNA)
- Stride
Unity is by far the most used of the C# engines and Godot is quickly gaining in popularity. Both have a massive community and it's fairly easy to get your problems fixed, their documentation is also very good.
Unity uses an alternative runtime for C# called Mono, it lacks certain of the latest features of C# and is typically slower than .NET, but the IL2CPP backend and the Burst compiler can improve performance. Unity is also paid (but free for small projects), whilst Godot is free, open source and uses the official C# runtime.
What makes C# fun and easy to write is also what makes it slower in a game setting, C# allocates a lot of objects into memory, which then need to be cleaned up by the garbage collector. The C# you write in game development tends to be very different than the C# you would write in a web or app setting. You need to be careful not to create long call chains on objects and instead cache the results, you can't use LINQ and the async pattern cannot be used as the primary model. There are a lot of considerations when building games and will be further explained in another post.
Entity Framework
There is a lot to say on this subject, but I'll keep it brief, EF Core is an Object Relational Mapper that maps your C# classes to SQL database tables and your lambda expressions into valid SQL queries. It is highly performant and supports major databases, such as:
- MS Sql Server
- Oracle
- MySQL
- PostgreSQL
- MongoDB (experimental)
- SQLite
EF Core enables rapid application development as deprecates the need for the repository pattern. It is performant, fun to write and easy to use and you can migrate from raw SQL using the included mapper.
Conclusion
C# and the .NET ecosystem is a very rich and mature ecosystem that makes coding fun while maintaining high performance. Having proper type safety at both runtime and compile time, detailed errors, multitudes of use cases, async, a huge integrated class library, multiple compilation options all point towards a mature and omnirole high-level language.
If you liked my content don't hesitate to check out DigitalOcean with my link or click on the image below, it would help us tremendously. They provide top-class hosting for cheap and won't break the bank, we host managed databases with them and had no issues so far DigitalOcean referral link
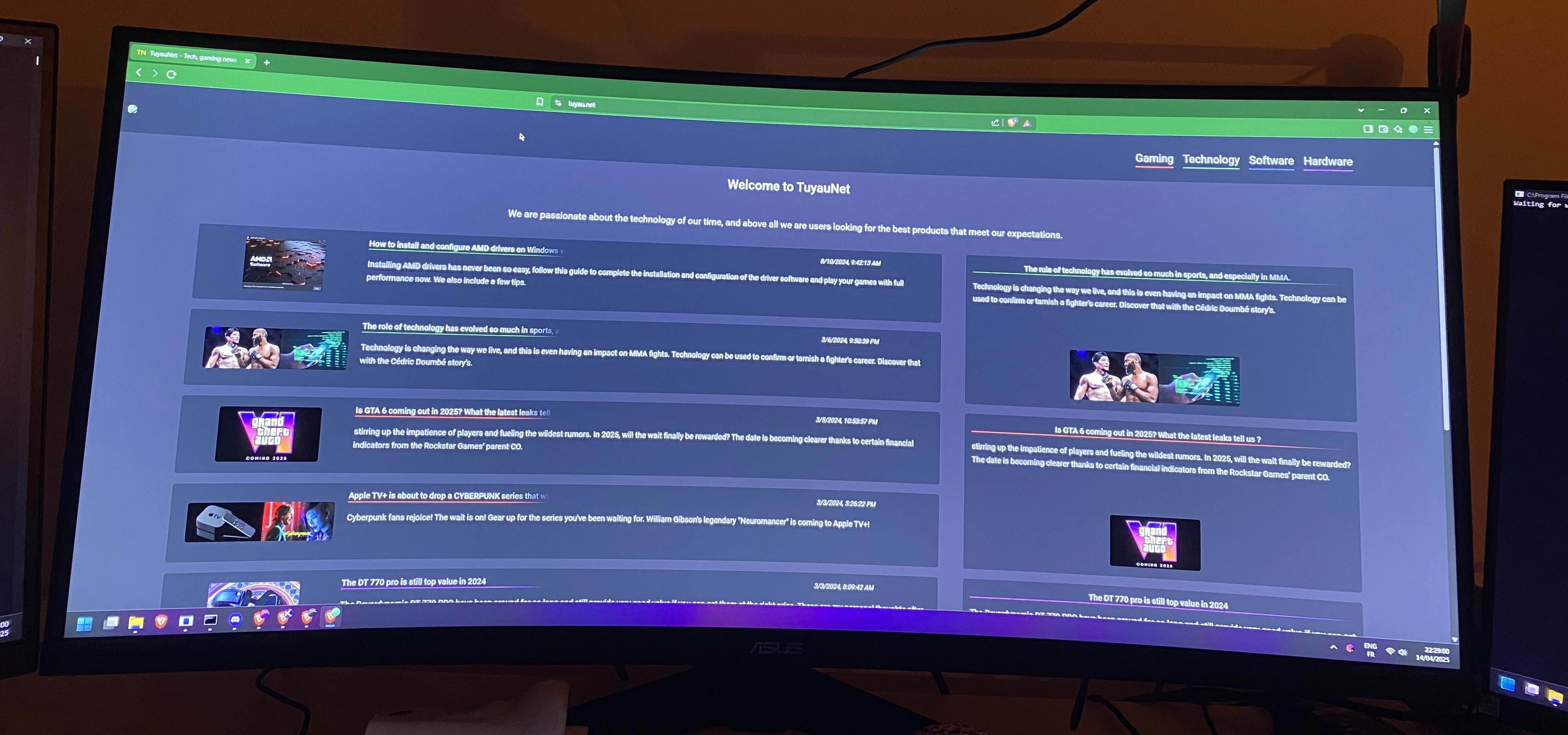
Technology is changing the way we live, and this is even having an impact on MMA fights. Technology can be used to confirm or tarnish a fighter's career. Discover that with the Cédric Doumbé story's.
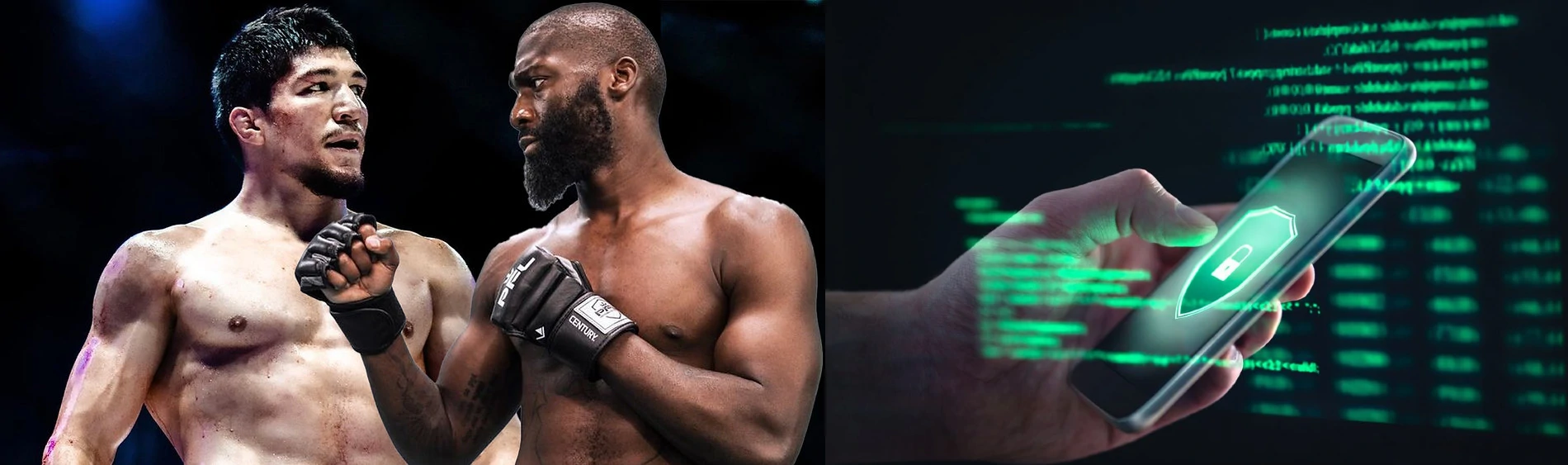
stirring up the impatience of players and fueling the wildest rumors. In 2025, will the wait finally be rewarded? The date is becoming clearer thanks to certain financial indicators from the Rockstar Games’ parent CO.
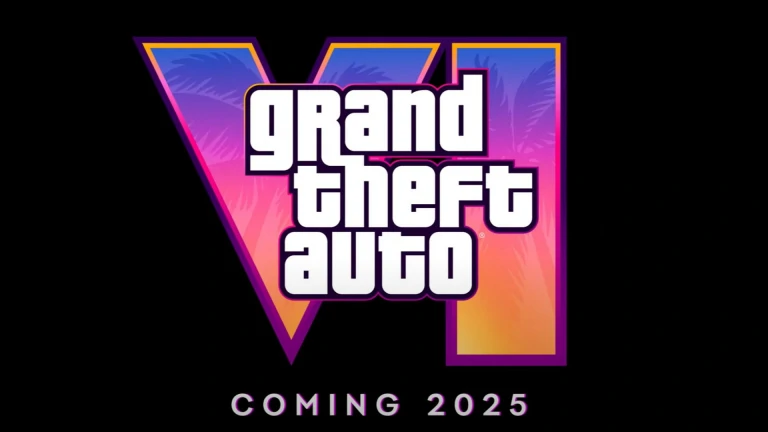
The Beyerdynamic DT 770 PRO have been around for so long and still provide very good value if you can get them at the right price. These are my personal thoughts after using them for over a year.
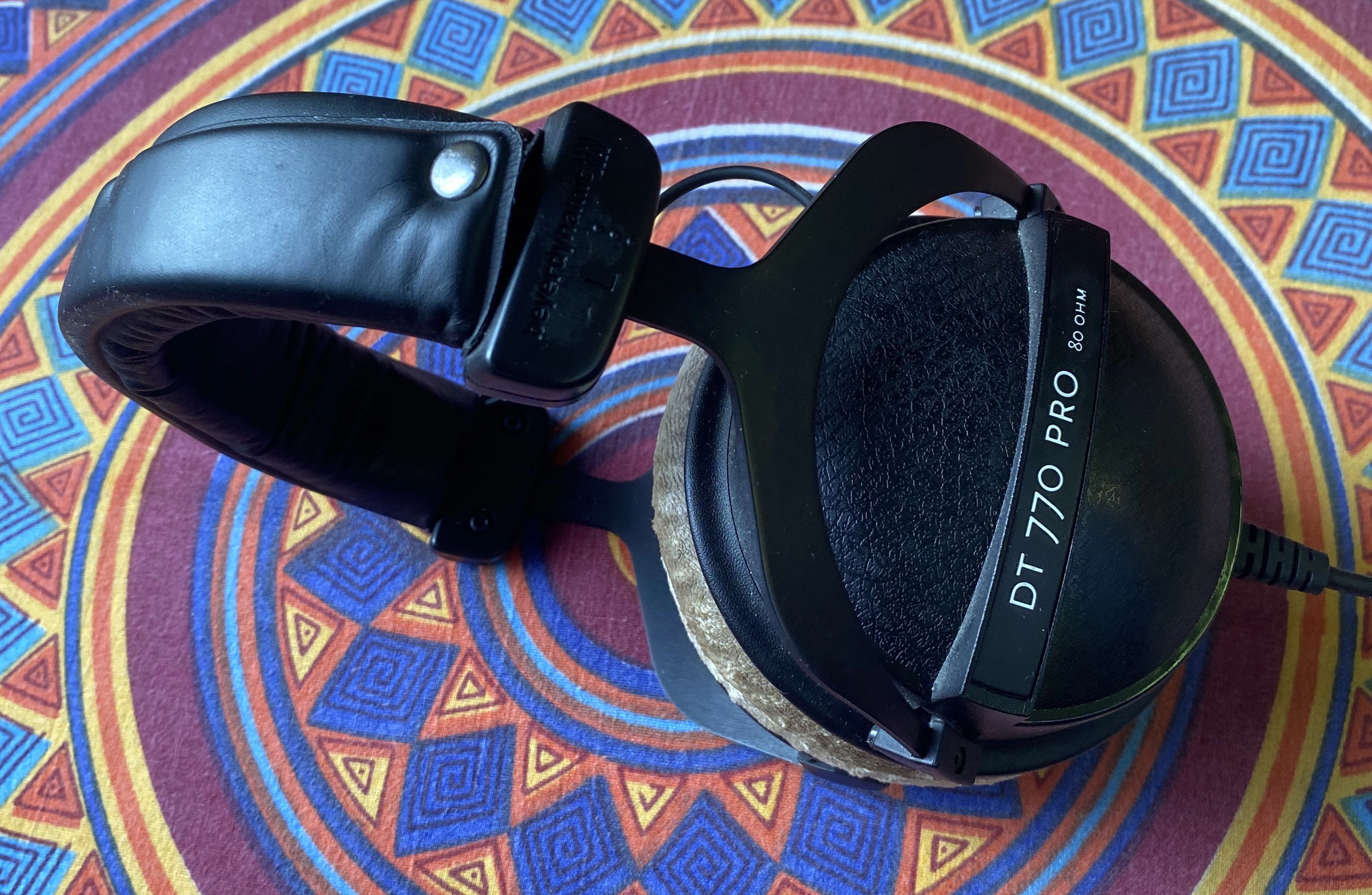
WARNO is a realistic cold war gone hot RTS which takes place in the 90s. Create your army and deploy it on the battlefield in casual 10v10 or competitive 1v1. It's insanely fun. Read more for the review.
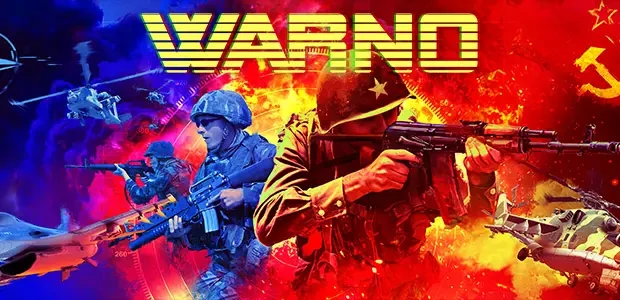
Activision's recent decisions regarding CALL OF DUTY have taken the game in directions that have sometimes been poorly received by the community. Is MODERN WARFARE 3 good at the moment?
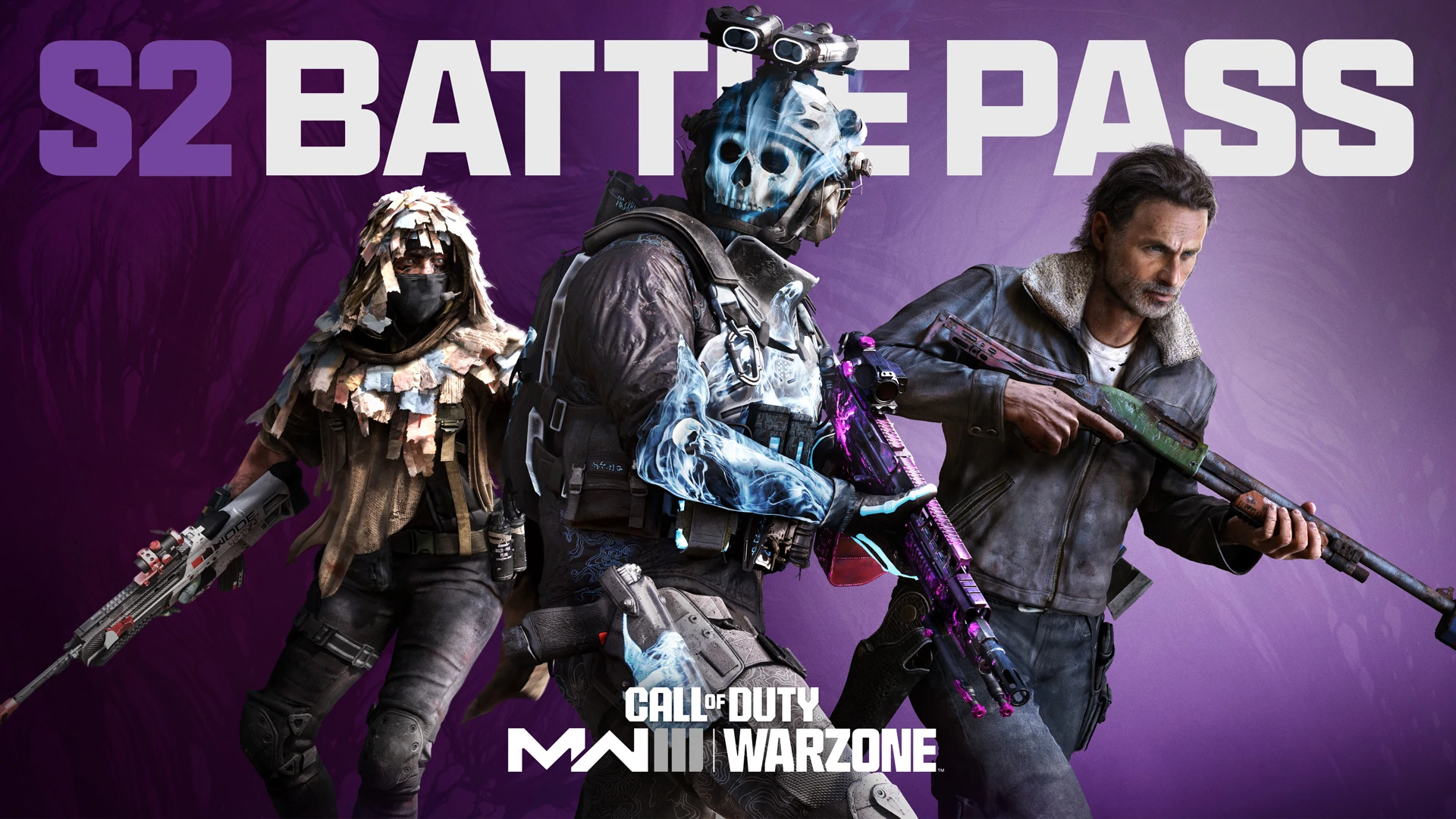
High-end gaming as been a luxury lately and we try to give you the best suggestions to achieve amazing performance in games for good prices. AMD vs NVidia have close competition in the mid-range and we dive into it.
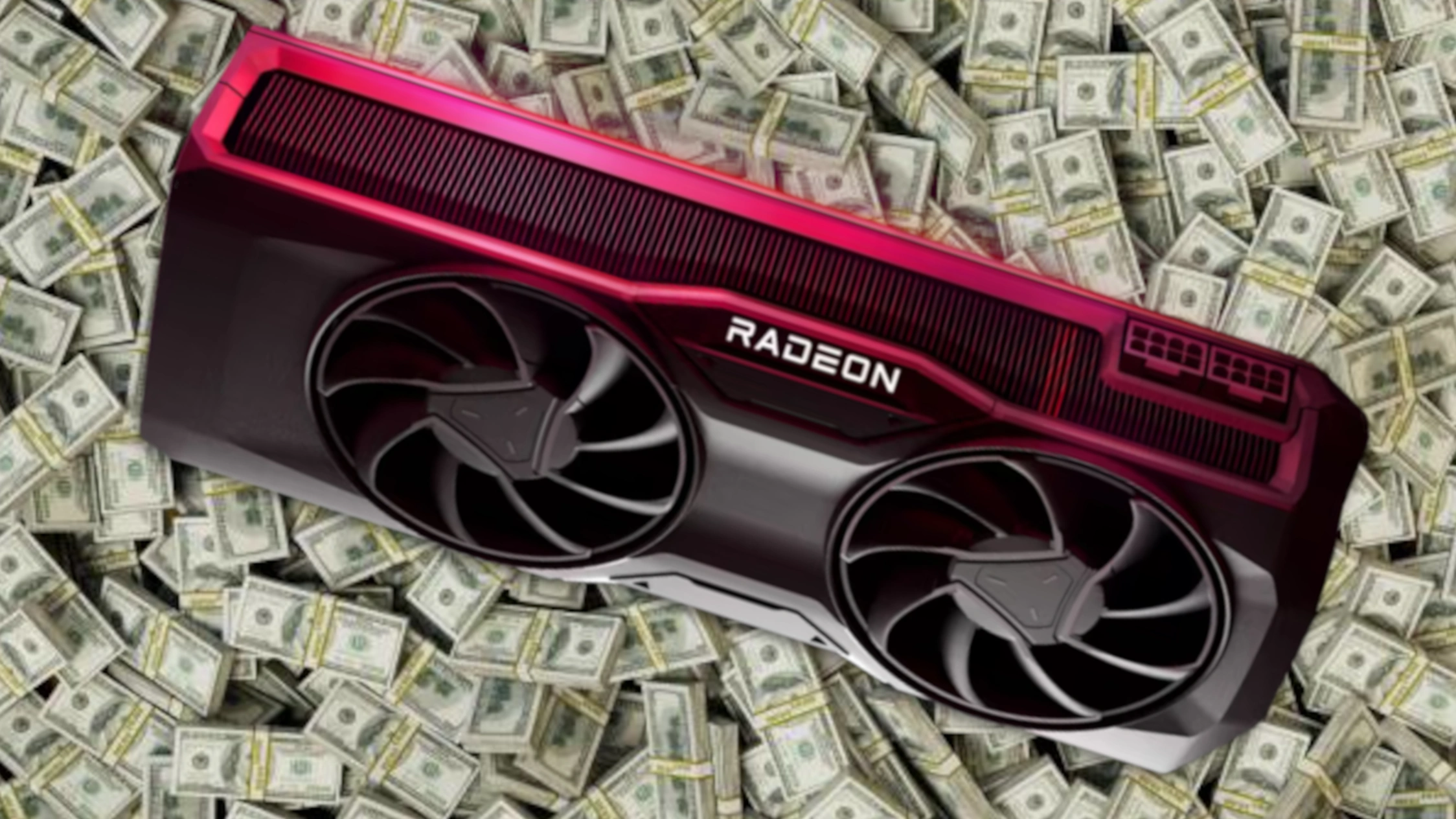
Finding the perfect cloud can be tricky, and if you make the wrong choice: very expensive! Read more about our take on the major cloud providers and how to avoid falling down an unfortunate pit.
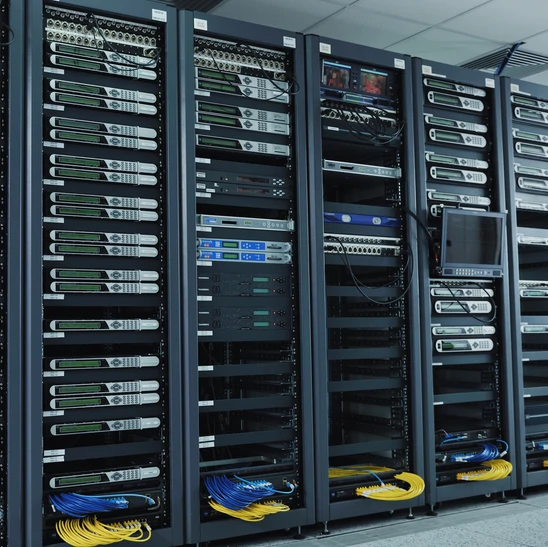
TEKKEN 8 The long awaited fighting game that will make you unleash your inner rage.
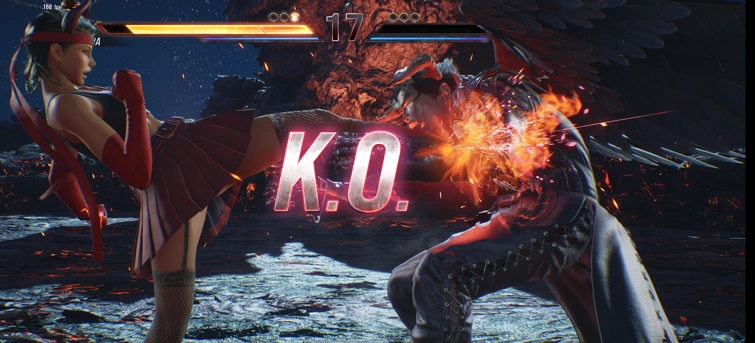
C# has enabled multitudes of performant and reliable applications to run for decades. A mature ecosystem, a huge class library and fun to write syntax make it a solid contender for the top league.
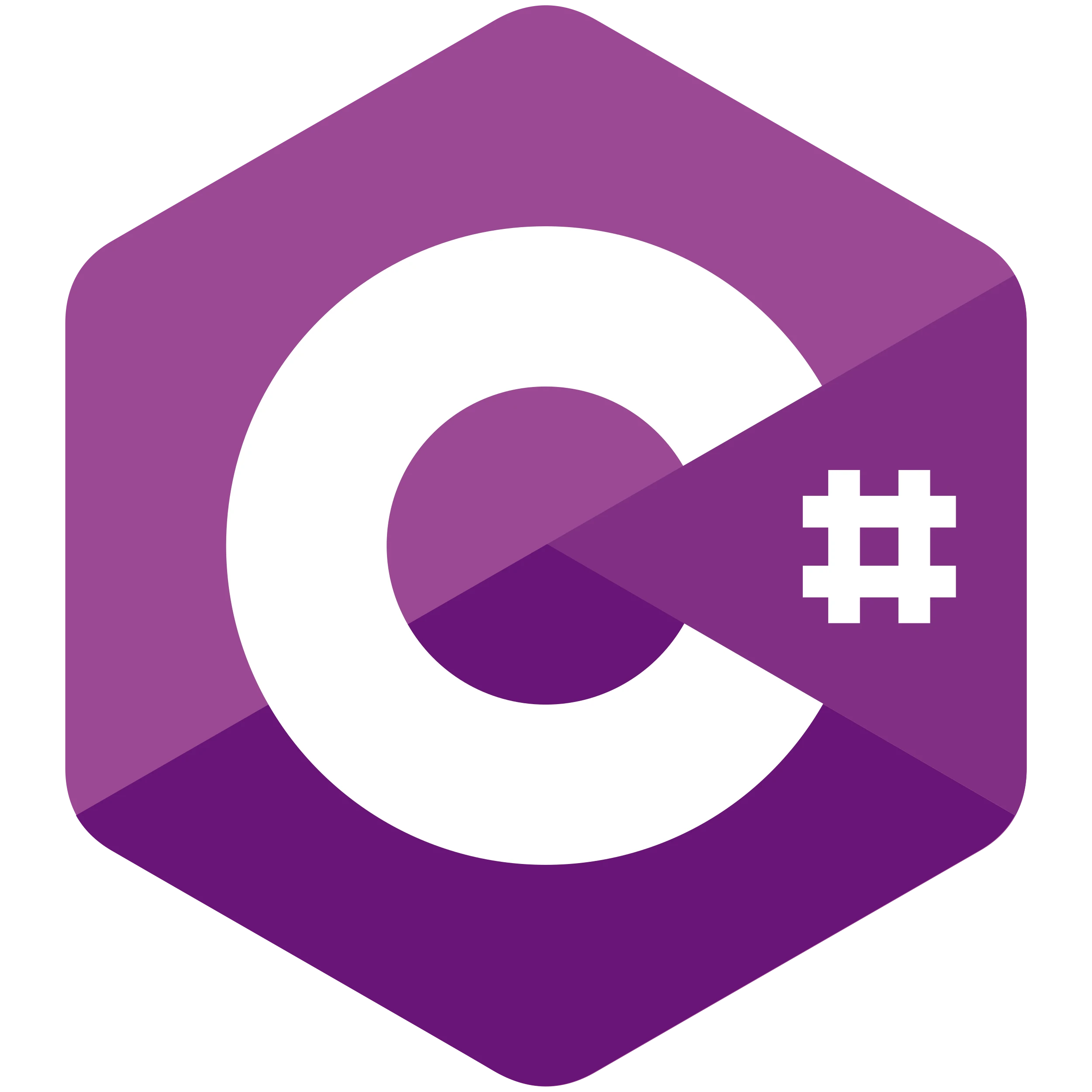
At Entity Systems we strive to bring you the best quality, fastest and most reliable software. From websites to mobile and desktop apps, all is possible. Read a bit more about us in this post!
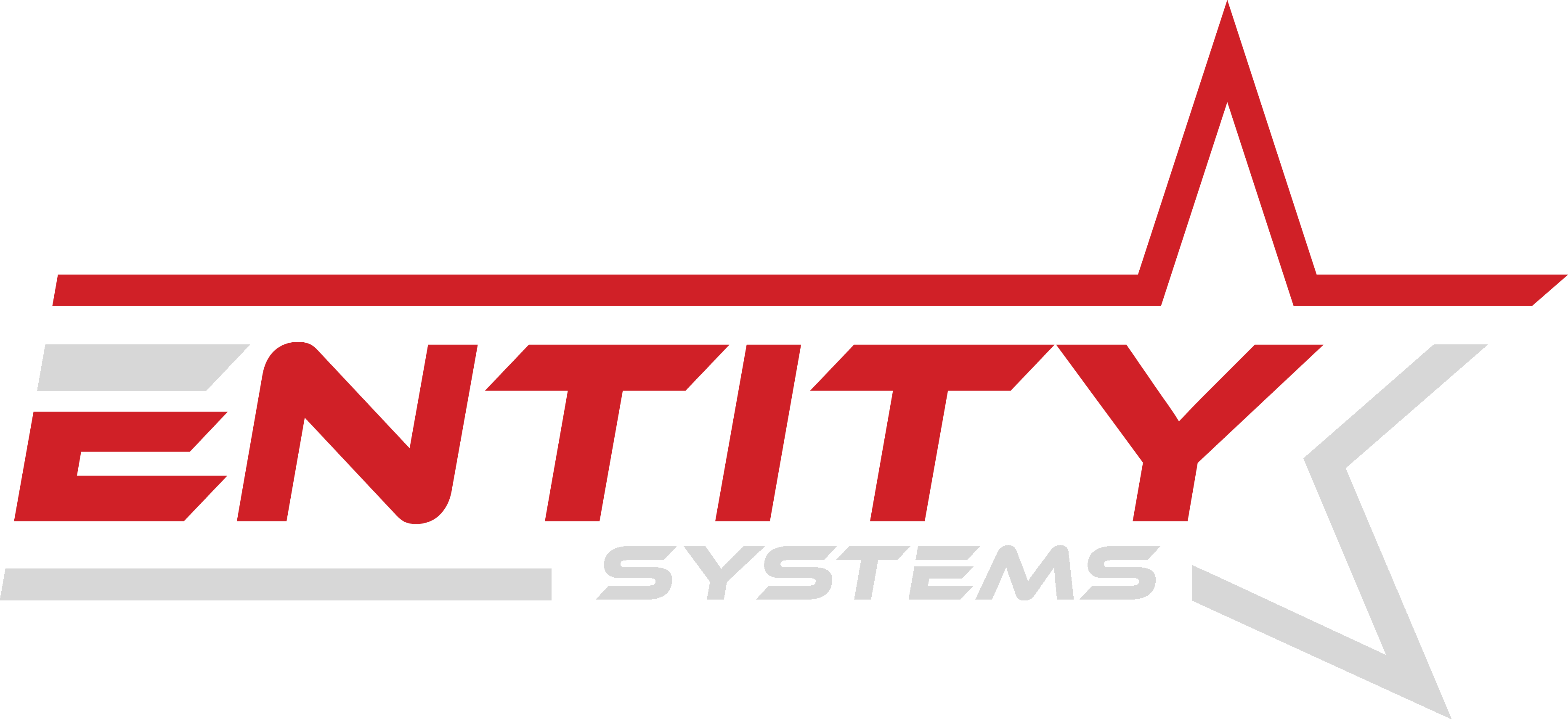